An array is a data structure representing an ordered collection of indexed items.
A common operation performed on multiple arrays is merge — when 2 or more arrays are merged to form a bigger array containing all the items of the merged arrays.
We can use the ES6 spread operator to merge different arrays into one:
const a = [1, 2, 3]; const b = [4, 5, 6]; const c = [7, 8, 9]; const final = [...a, ...b, ...c]; // [1, 2, 3, 4, 5, 6, 7, 8, 9]
The spread operator is handy if we use the `push`
method as well:
const a = [1, 2, 3]; const b = [4, 5, 6]; a.push(...b); a; // [1, 2, 3, 4, 5, 6]
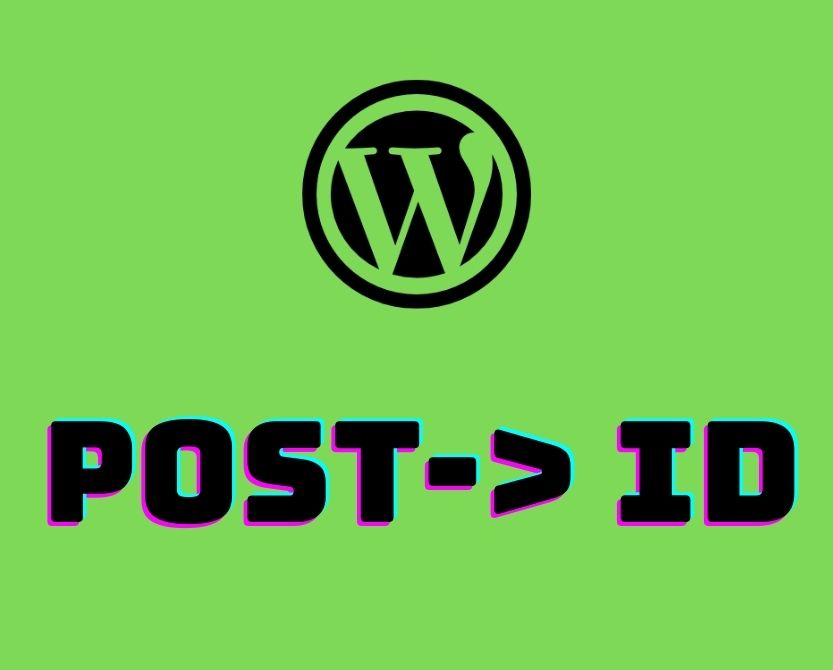
Previous Article